Creative SB0350 Hardware Programming Guide - Page 25
Creative SB0350 Manual
![]() |
View all Creative SB0350 manuals
Add to My Manuals
Save this manual to your list of manuals |
Page 25 highlights
Introduction to DSP Programming 2-3 Sound Blaster card may not be installed or an incorrect I/O address is being used. You should exit the reset process and declare an error. The following assembly code fragment shows the process of resetting the DSP: mov add mov out sub Delay: dec jnz out sub Empty: mov add in or jns sub in cmp je dx,wSBCBaseAddx dl,6 al,1 dx,al al,al al Delay dx,al cx,cx dx,wSBCBaseAddx dl,0Eh al,dx al,al NextAttempt dl,4 al,dx al,0AAh ResetOK ; SBC base I/O address 2x0h ; Reset port, 2x6h ; Write a 1 to the DSP reset port ; ; Delay loop ; Write a 0 to the DSP reset port ; Maximum of 65536 tries ; SBC base I/O address 2x0h ; Read-Buffer Status port, 2xEh ; Read Read-Buffer Status port ; Data available? ; Bit 7 clear, try again ; ; ; ; Read Data port, 2xAh Read in-bound DSP data Receive success code, 0AAh? SUCCESS! NextAttempt: loop Empty ; Try again ;*** ;*** Failed to reset DSP: Sound Blaster not detected! Reading from DSP When DSP data is available, it can be read in from the Read Data port. Before the data is read in, bit-7 of the Read-Buffer Status port must be checked to ensure that there is data to read. If bit-7 is 1, then there is data to read. Otherwise, no data is available. The following assembly code fragment shows the process of reading data from the DSP: mov add Busy: in or jns sub in al,dx al,al Busy dl,4 al,dx ; Check for in-bound data ; Data available? ; Bit 7 clear, try again ; Read Data port, 2xAh ; Read in-bound DSP data dx,wSBCBaseAddx dl,0Eh ; SBC base I/O address 2x0h ; Read-Buffer Status port, 2xEh
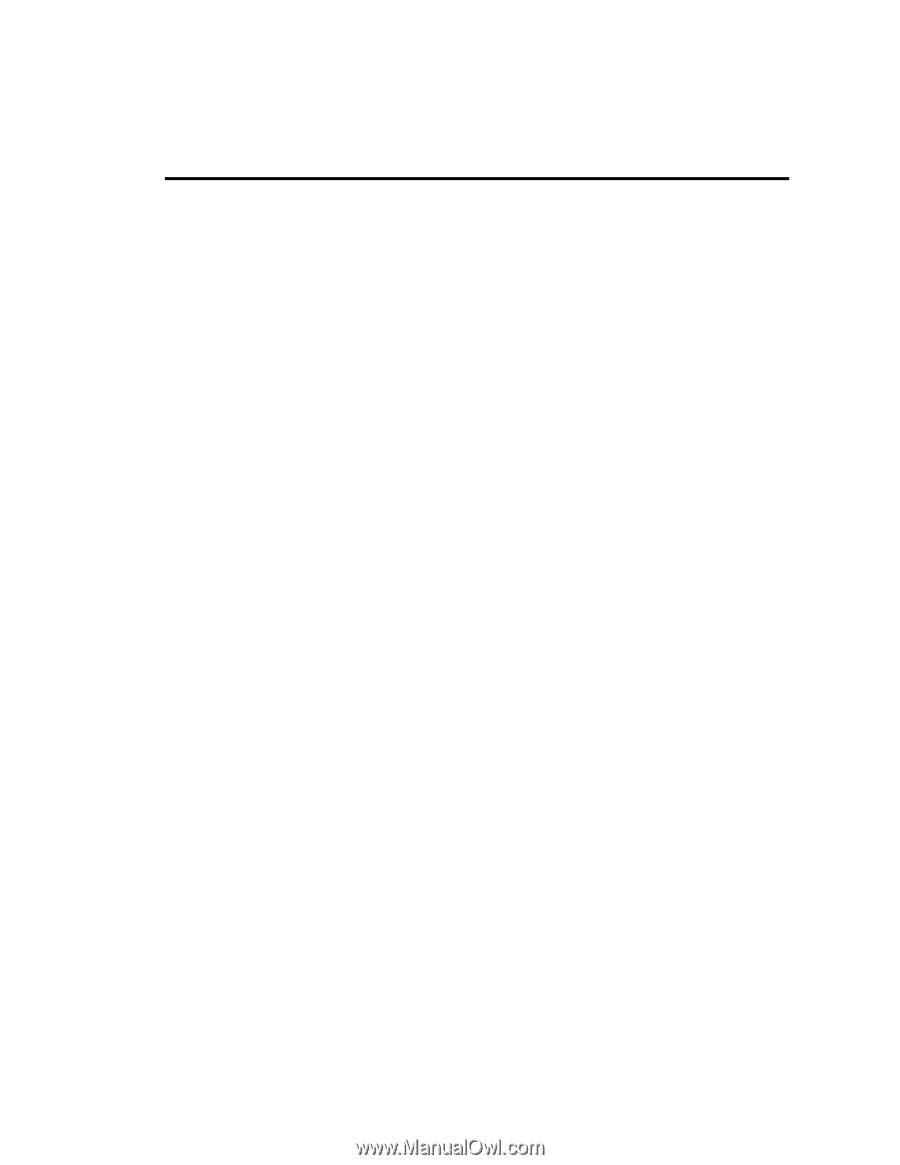