Seagate ST3500630A Serial ATA Native Command Queuing (670K, PDF) - Page 9
Using Asynchronous I/O in Windows
![]() |
UPC - 000067575145
View all Seagate ST3500630A manuals
Add to My Manuals
Save this manual to your list of manuals |
Page 9 highlights
Serial ATA Native Command Queuing an event to be signaled or by receiving a callback. Since the call returns immediately, the application can continue to do useful work, including issuing more read or write file functions. The preferred method for writing an application that needs to make several different file accesses is to issue all of the file accesses using non-blocking I/O calls. Then the application can use events or callbacks to determine when individual calls have completed. If there are a large number of I/Os, on the order of four to eight, by issuing all of the I/Os at the same time the total time to retrieve all of the data can be cut in half. Using Asynchronous I/O in Windows* In Windows* applications, there are two main functions used for accessing files called ReadFile and WriteFile. In a typical application, these functions are used in a blocking, or synchronous, manner. An example is shown below of reading 1KB from a file using ReadFile: bStatus = ReadFile( hFile, pBuffer, 1024, &numBytesRead, NULL); // Handle to file to read from // Pointer to buffer to place data in // Want to read 1024 bytes from the file // Number of bytes read from the file // Synchronous so overlapped parameter is NULL // // Code for checking the status value and ensuring there were not any errors. // ... When this call is made, it does not return until all of the data is read from the file. During this time, the application cannot do any useful work nor can it issue any more I/O calls within this thread. A preferred method for reading data from a file is to use ReadFile and WriteFile in an asynchronous manner by opening the file using the flag FILE_FLAG_OVERLAPPED. The same example is shown below using the asynchronous mechanism. // // Fill in the OVERLAPPED structure used for asynchronous IO. // overlap.offset = 0; // Offset in the file to start reading from overlap.offsetHigh = 0; // Upper 32-bits of offset overlap.hEvent = hEvent; // Event to trigger when complete, initialized // using CreateEvent() // // Make the call to start reading the file. // bStatus = ReadFile( hFile, // Handle to file to read from pBuffer, // Pointer to buffer to place data in 1024, // Want to read 1024 bytes from the file &numBytesRead, // Number of bytes read from the file 9 *Other names and brands may be claimed as the property of others.
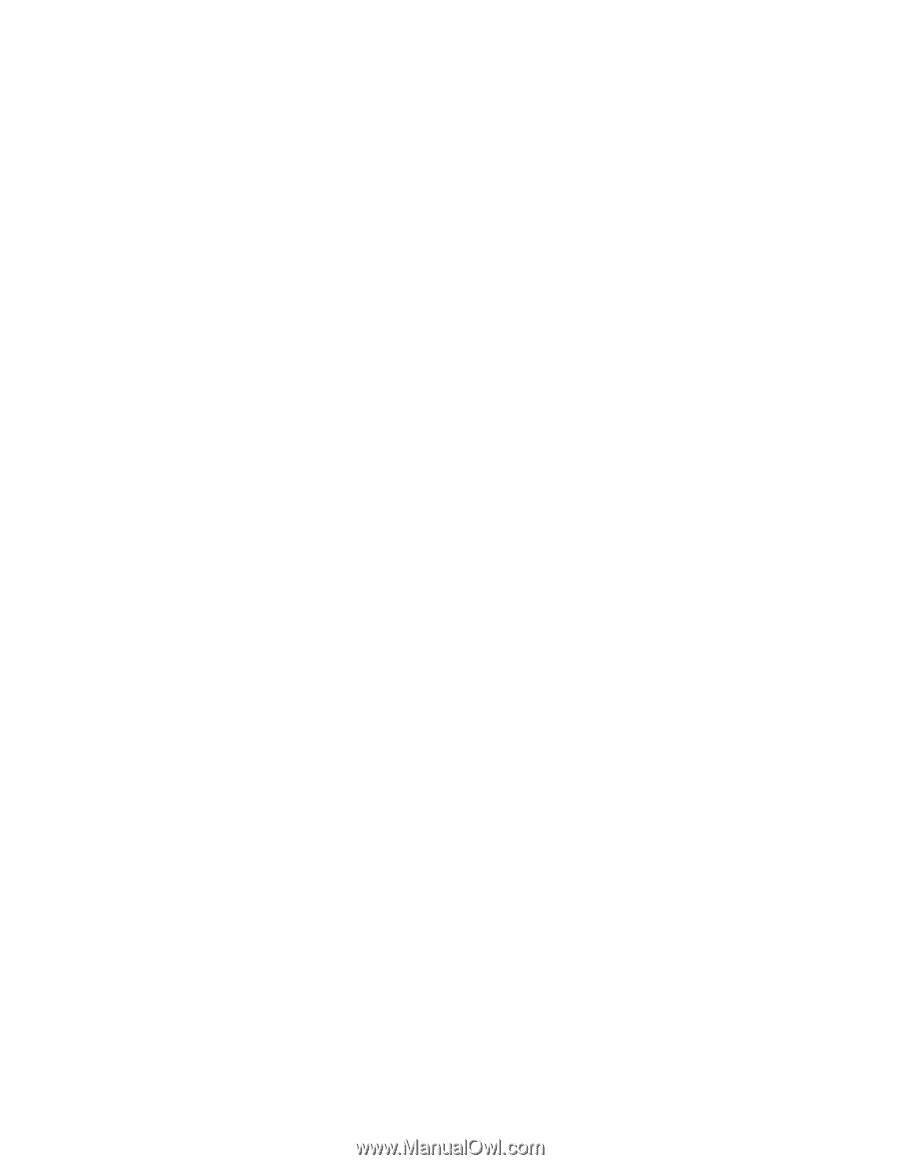