HP 800 HP DLPI Programmer's Guide - Page 184
ioctl Example, Sample Programs
![]() |
View all HP 800 manuals
Add to My Manuals
Save this manual to your list of manuals |
Page 184 highlights
Sample Programs ioctl Example ioctl Example (C) COPYRIGHT HEWLETT-PACKARD DEVELOPMENT COMPANY L.P. 2003. ALL RIGHTS RESERVED. NO PART OF THIS PROGRAM MAY BE PHOTOCOPIED, REPRODUCED, OR TRANSLATED TO ANOTHER PROGRAM LANGUAGE WITHOUT THE PRIOR WRITTEN CONSENT OF HEWLETT PACKARD COMPANY The main part of this program is composed of two parts. The first part demonstrates data transfer over a connectionless stream with LLC SAP headers. The second part of this program demonstrates data transfer over a connectionless stream with LLC SNAP headers #include #include #include #include #include #include #defineAREA_SIZE1000/* Area size */ char buf_ctl[AREA_SIZE]; /* for control messages */ struct strbuf ctlbuf = { AREA_SIZE,/* maxlen = AREA_SIZE */ 0,/* len gets filled in for each message */ buf_ctl/* buf = control area */ }; extern int errno; /* Options that are handled by this program */ #defineGET_MTU 0 /* Get MTU */ #defineSET_MTU 1/* Set MTU */ Routine that will issue a DL_HP_GET_DRV_PARAM_IOCTL to the PPA that 184 Appendix A
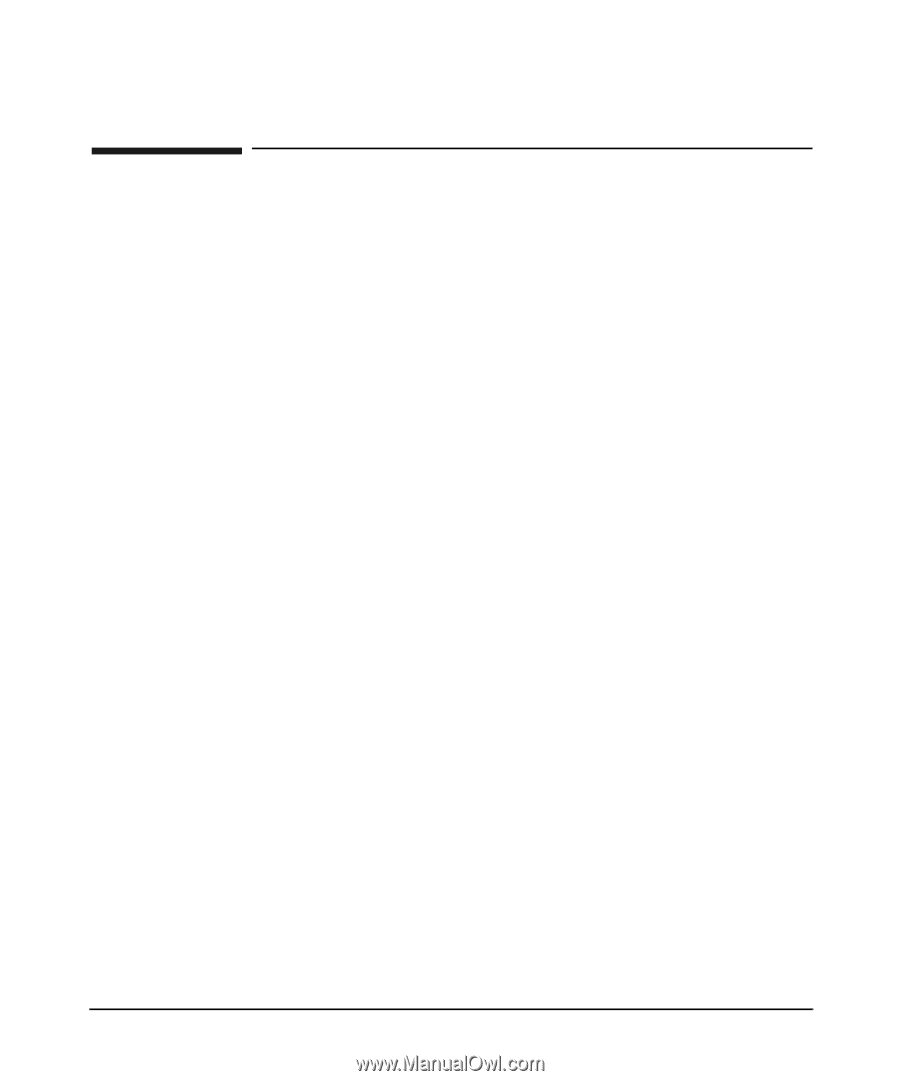