HP 800 HP DLPI Programmer's Guide - Page 189
Connection Mode Example, define LONG_AREA_SIZEAREA_SIZE / sizeofu_long /* AREA_SIZE / 4
![]() |
View all HP 800 manuals
Add to My Manuals
Save this manual to your list of manuals |
Page 189 highlights
Sample Programs Connection Mode Example Connection Mode Example B.11.23_ic60 (C) COPYRIGHT HEWLETT-PACKARD DEVELOPMENT COMPANY L.P. 2003. ALL RIGHTS RESERVED. NO PART OF THIS PROGRAM MAY BE PHOTOCOPIED, REPRODUCED, OR TRANSLATED TO ANOTHER PROGRAM LANGUAGE WITHOUT THE PRIOR WRITTEN CONSENT OF HEWLETT PACKARD COMPANY This program demonstrates data transfer over a connection oriented DLPI stream. It also demonstrates connection handoff #include #include #include #include #include #include #include #include #define SEND_SAP #define RECV_SAP 0x80 0x82 /* sending SAP */ /* receiving SAP */ global areas for sending and receiving messages define AREA_SIZE 5000/* bytes; big enough for largest possible msg */ #define LONG_AREA_SIZE(AREA_SIZE / sizeof(u_long)) /* AREA_SIZE / 4 */ /* these are u_long arrays instead of u_char to insure proper alignment */ u_longctrl_area[LONG_AREA_SIZE];/* for control messages */ u_longdata_area[LONG_AREA_SIZE];/* for data messages */ struct strbuf ctrl_buf = { AREA_SIZE,/* maxlen = AREA_SIZE */ 0,/* len gets filled in for each message */ ctrl_area/* buf = control area */ }; Appendix A 189
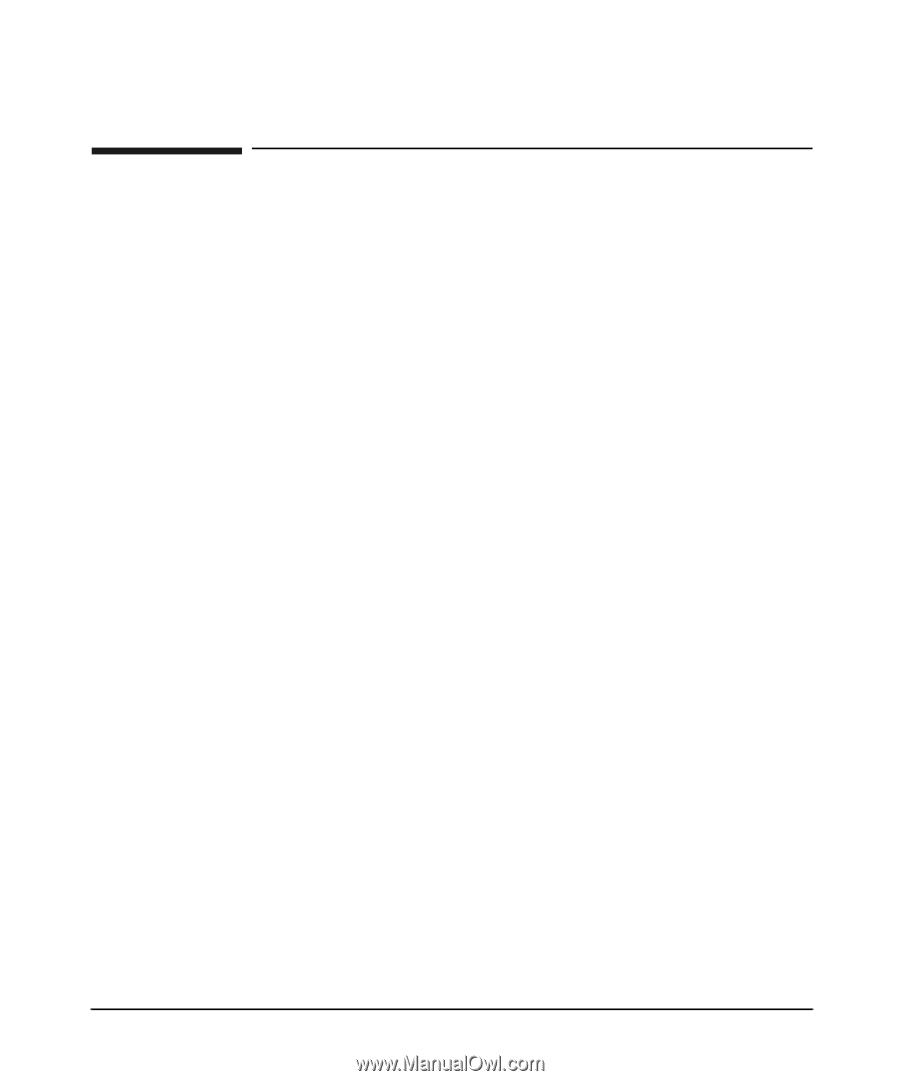