HP Workstation zx2000 HP OpenGL Implementation Guide for HP-UX 11.X (IPF versi - Page 38
state changes and their effects on display lists, regular primitive data, GL_TRIANGLES
![]() |
View all HP Workstation zx2000 manuals
Add to My Manuals
Save this manual to your list of manuals |
Page 38 highlights
programming hints state changes and their effects on display lists If there are several state changes in a row, it is possible, in some circumstances, for the display list to optimize them. It is more efficient to put a state change before a glBegin, than after it. For example, this is always more efficient: glColor3f(1,2,3); glBegin(GL_TRIANGLES); glVertex3f(...); ... many more vertices... glEnd(); than this: glBegin(GL_TRIANGLES); glColor3f(1,2,3); glVertex3f(...); ... many more vertices... glEnd(); For performance efficiency avoid glMaterial state changes, especially within a glBegin/glEnd pair. regular primitive data If the vertex data that you give to a display list is regular (that is, every vertex has the same data associated with it), it is possible for the display list to optimize the primitive much more effectively than if the data is not regular. For example if you wanted to give only a single normal for each face in a GL_TRIANGLES primitive, the most intuitive way to get the best performance would look like this: glBegin(GL_TRIANGLES); glNormal3fv(&v1); glVertex3fv(&p1); glVertex3fv(&p2); glVertex3fv(&p3); glNormal3fv(&v2); glVertex3fv(&p4); glVertex3fv(&p5); glVertex3fv(&p6); ... glEnd(); In immediate mode, this would give you the best performance. However, if you are putting these calls into a display list, you will get much better performance by duplicating the normal for each vertex, thereby giving regular data to the display list: glBegin(GL_TRIANGLES); glNormal3fv(&v1); glVertex3fv(&p1); glNormal3fv(&v1); glVertex3fv(&p2); glNormal3fv(&v1); glVertex3fv(&p3); glNormal3fv(&v2); glVertex3fv(&p4); glNormal3fv(&v2); glVertex3fv(&p5); glNormal3fv(&v2); glVertex3fv(&p6); ... glEnd(); The reason this is faster is the display list can optimize this type of primitive into a single, very efficient structure. The small cost of adding extra data is offset by this optimization. 5-4 OpenGL implementation guide
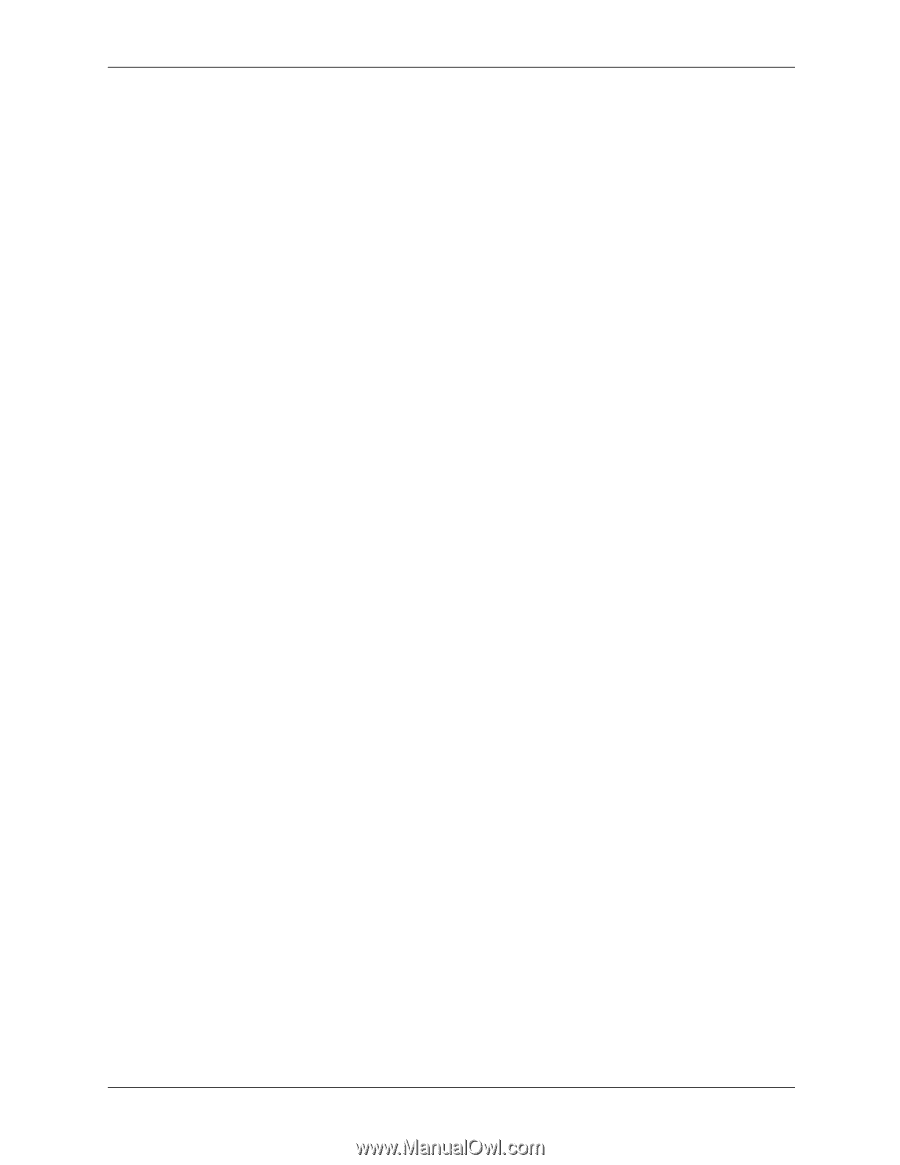