Adobe 0046100128056 Scripting Guide - Page 199
Applying formatting with XML rules
![]() |
UPC - 718659087562
View all Adobe 0046100128056 manuals
Add to My Manuals
Save this manual to your list of manuals |
Page 199 highlights
CHAPTER 13: XML Rules XML Rules Examples 199 Applying formatting with XML rules The previous XML-rule examples have shown basic techniques for finding XML elements, rearranging the order of XML elements, and adding text to XML elements. Because XML rules are part of scripts, they can perform almost any action-from applying text formatting to creating entirely new page items, pages, and documents. The following XML-rule examples show how to apply formatting to XML elements using XML rules and how to create new page items based on XML-rule matching. The following script adds static text and applies formatting to the example XML data (for the complete script, see XMLRulesApplyFormatting): main(); function main(){ if (app.documents.length != 0){ var myDocument = app.documents.item(0); //Document set-up. myDocument.viewPreferences.horizontalMeasurementUnits = MeasurementUnits.points; myDocument.viewPreferences.verticalMeasurementUnits = MeasurementUnits.points; myDocument.colors.add({model:ColorModel.process, colorValue:[0, 100, 100, 0], name:"Red"}); myDocument.paragraphStyles.add({name:"DeviceName", pointSize:24, leading:24, spaceBefore:24, fillColor:"Red", paragraphRuleAbove:true}); myDocument.paragraphStyles.add({name:"DeviceType", pointSize:12, fontStyle:"Bold", leading:12}); myDocument.paragraphStyles.add({name:"PartNumber", pointSize:12, fontStyle:"Bold", leading:12}); myDocument.paragraphStyles.add({name:"Voltage", pointSize:10, leading:12}); myDocument.paragraphStyles.add({name:"DevicePackage", pointSize:10, leading:12}); myDocument.paragraphStyles.add({name:"Price", pointSize:10, leading:12, fontStyle:"Bold"}); var myRuleSet = new Array (new ProcessDevice, new ProcessName, new ProcessType, new ProcessPartNumber, new ProcessSupplyVoltage, new ProcessPackageType, new ProcessPackageOne, new ProcessPackages, new ProcessPrice); with(myDocument){ var elements = xmlElements; __processRuleSet(elements.item(0), myRuleSet); } } else{ alert("No open document"); } function ProcessDevice(){ this.name = "ProcessDevice"; this.xpath = "/devices/device"; this.apply = function(myElement, myRuleProcessor){ with(myElement){ insertTextAsContent("\r", XMLElementPosition.afterElement); } return true; }
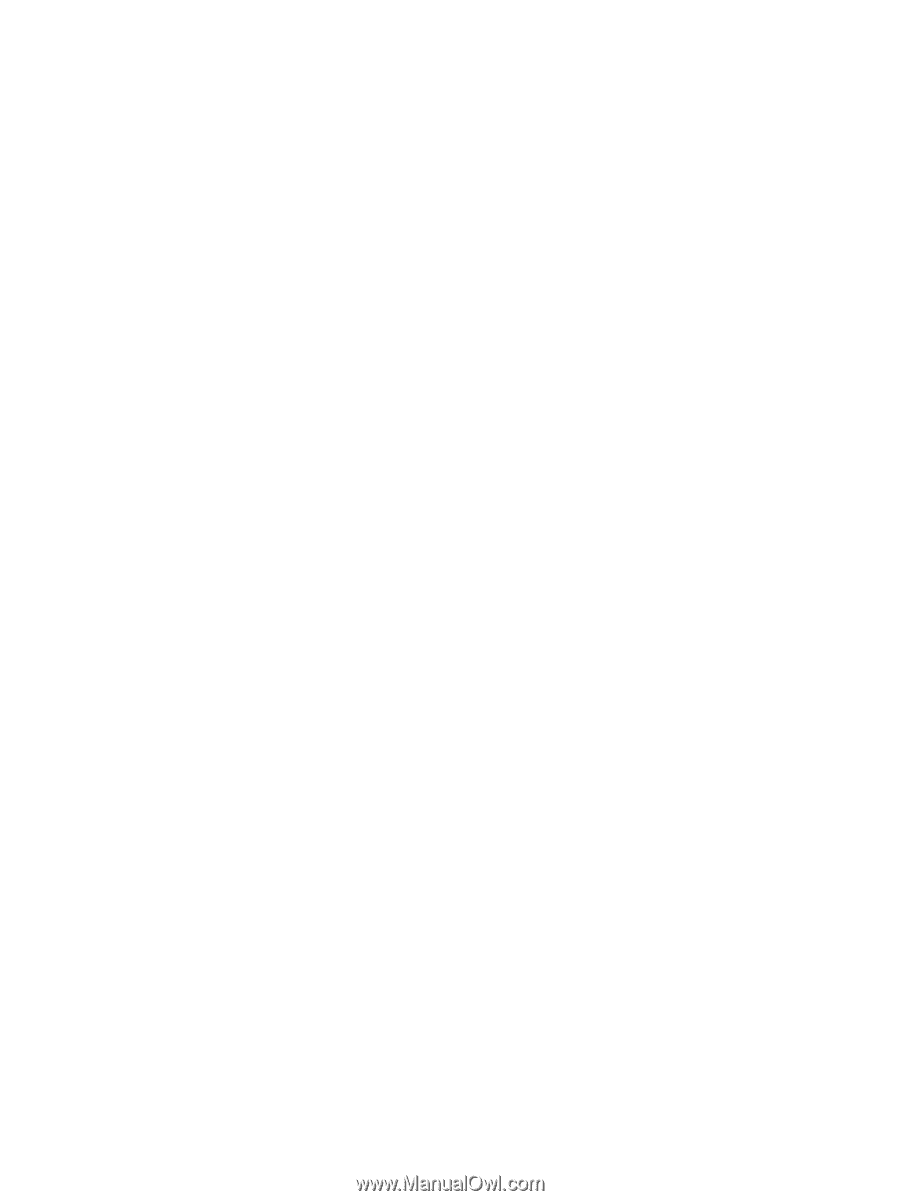