HP Dx9000 Resources for Developing Touch-Friendly Applications for HP Business - Page 11
Enabling/Disabling Touch Pointer
![]() |
UPC - 884420541578
View all HP Dx9000 manuals
Add to My Manuals
Save this manual to your list of manuals |
Page 11 highlights
} private void inkCanvas_OnGesture(object sender, InkCanvasGestureEventArgs e) { ApplicationGesture gesture = e.GetGestureRecognitionResults()[0].ApplicationGesture; if (gesture == ApplicationGesture.Triangle || gesture == ApplicationGesture.Square || gesture == ApplicationGesture.Circle) { //remove the stroke on the ink canvas StrokeCollection strokesToDelete = inkCanvas.Strokes.HitTest(e.Strokes.GetBounds(), 10); inkCanvas.Strokes.Remove(strokesToDelete); //do some action here } else { //not the expected gestures e.Cancel = true; } } Enabling/Disabling Touch Pointer The touch pointer is the Windows floating graphic that looks like a mouse on screen. This pointer helps you target small objects since touching small targets is not accurate for a finger. To show the touch pointer when touching the screen, click Control Panel > Pen and Input Devices > Touch tab > Make sure Show the touch pointer when I'm interacting with items on the screen. Click Apply. If you need to disable and/or enable the touch pointer in your code, you can intercept operating system window messages using a window procedure (WndProc) and modify the Windows messages. The C# pseudo-code below shows how to enable and disable the touch pointer: const int WM_TABLET_QUERY_SYSTEM_GESTURE_STATUS = 716; const uint SYSTEM_GESTURE_STATUS_TOUCHUI_FORCEON = 0x00000100; const uint SYSTEM_GESTURE_STATUS_TOUCHUI_FORCEOFF = 0x00000200; protected override void WndProc(ref Message msg) { switch (msg.Msg) { case WM_TABLET_QUERY_SYSTEM_GESTURE_STATUS: 11
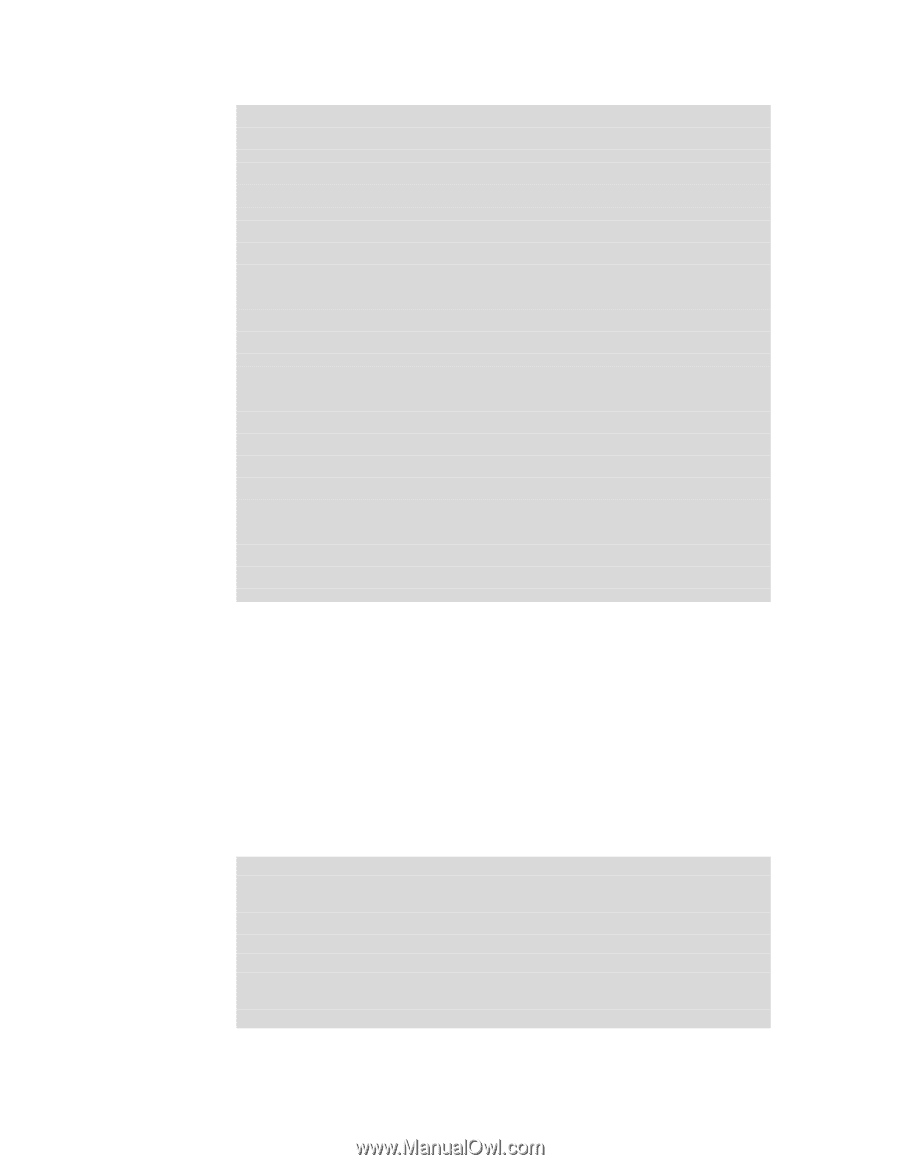